How To Add Multiple Pages in React (2023 Tutorial)
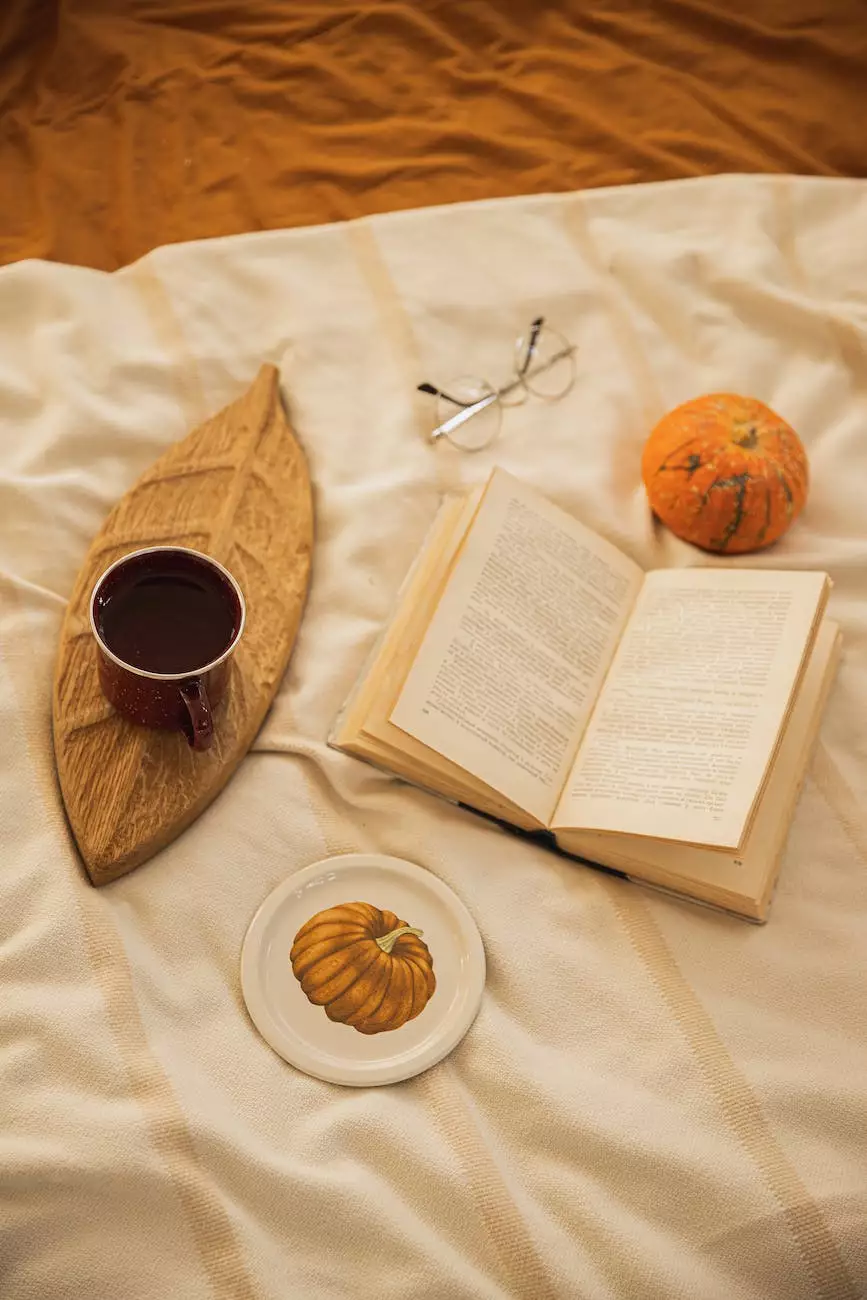
Welcome to our tutorial on how to add multiple pages in React! In this comprehensive guide, we will provide you with all the information you need to effortlessly create multiple pages within your React application. Are you ready to take your React development skills to the next level? Let's dive right in!
Why Use Multiple Pages in React?
Before we get started on the implementation details, let's briefly discuss why it's important to use multiple pages in React. With single-page applications (SPAs) becoming more popular, it's easy to overlook the benefits of using multiple pages. However, there are several reasons why you might want to consider implementing multiple pages in your React application:
- Improved SEO: Having multiple pages allows search engines to index each page individually, potentially improving your website's search engine rankings.
- Better organization: With multiple pages, you can effectively separate different sections, features, or functionalities of your application, making it easier to manage and maintain.
- Enhanced user experience: Using multiple pages enables smoother navigation and a more familiar browsing experience for your users.
Getting Started
Now that you understand the advantages of using multiple pages in React, let's dive into the steps you need to follow to implement this feature in your own application.
Step 1: Install React Router
To create multiple pages in React, we will be using React Router, a widely-used routing library. Start by installing React Router using npm:
$ npm install react-router-domOnce the installation is complete, you can now import the necessary components and start defining your routes.
Step 2: Define Routes
With React Router installed, it's time to define the routes for your pages. In a nutshell, routes determine which component should be rendered based on the current URL. Let's take a look at an example:
{` import { BrowserRouter as Router, Route, Switch } from 'react-router-dom'; function App() { return ( ); }`}In this example, we use the `BrowserRouter` component as the parent container for our routes. Inside the `Switch` component, we define individual routes using the `Route` component, specifying the path and the corresponding component to render.
Step 3: Create Page Components
As seen in the previous code snippet, each route points to a specific component. Now it's time to create those components that will serve as your individual pages. Here's an example:
{` import React from 'react'; const Home = () => { return (Welcome to the Home Page
This is the main page of our application.
); }; const About = () => { return (About Us
Learn more about our company and values.
); }; const Contact = () => { return (Contact Us
Get in touch with our team for any inquiries.
); }; `}In this example, we have three components: `Home`, `About`, and `Contact`. Each component represents a separate page within your application and contains the relevant content for that page.
Step 4: Linking and Navigation
Now that you have your routes and page components set up, you need a way to navigate between them. React Router provides the `Link` component for this purpose. Here's an example:
{` import { Link } from 'react-router-dom'; function Navigation() { return (- Home
- About
- Contact
In this example, we use the `Link` component to create navigation links. Each link corresponds to a specific route defined earlier, allowing users to easily switch between pages.
Conclusion
Congratulations! You have successfully learned how to add multiple pages in React using React Router. By following the steps outlined in this tutorial, you can now enhance your applications with improved SEO, better organization, and a more seamless user experience.
Remember, implementing multiple pages in React can have a significant impact on your website's search engine rankings and overall user satisfaction. So go ahead, experiment with different page layouts, and take your React projects to new heights!
This tutorial was brought to you by Genevish Graphics, experts in Arts & Entertainment - Visual Arts and Design. Contact us today for all your creative needs!